How To: Get the size of a view in SwiftUI
Published on 19 Oct 2023
Here’s how you can get the size of any view in SwiftUI using the GeometryReader.
The Problem
You are trying to figure out what size a certain view is.
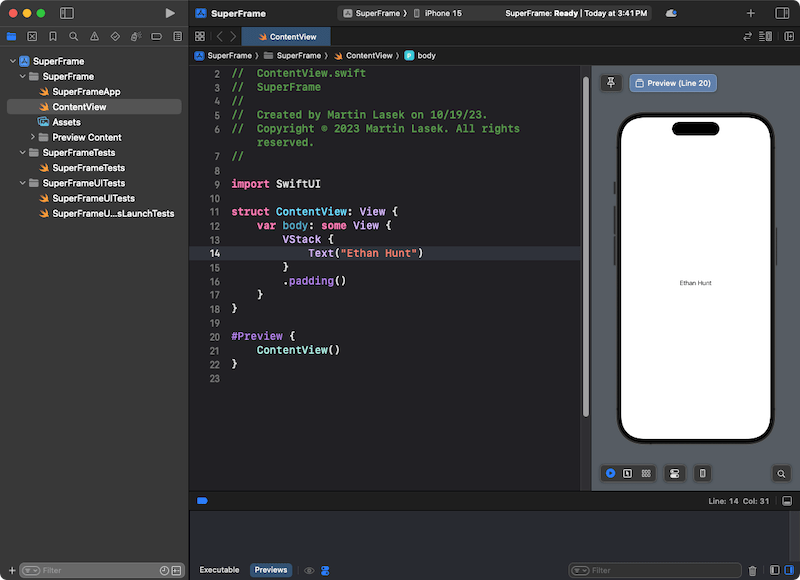
The Solution
struct ContentView: View {
var body: some View {
Text("Ethan Hunt")
.background(
GeometryReader { proxy in
Color.red.onAppear {
print("🔥 \(proxy.size)")
}
}
)
}
}
We are using the background modifier to fill it with the color red. By wrapping our color in a GeometryReader we are able to read the size of that color plane.
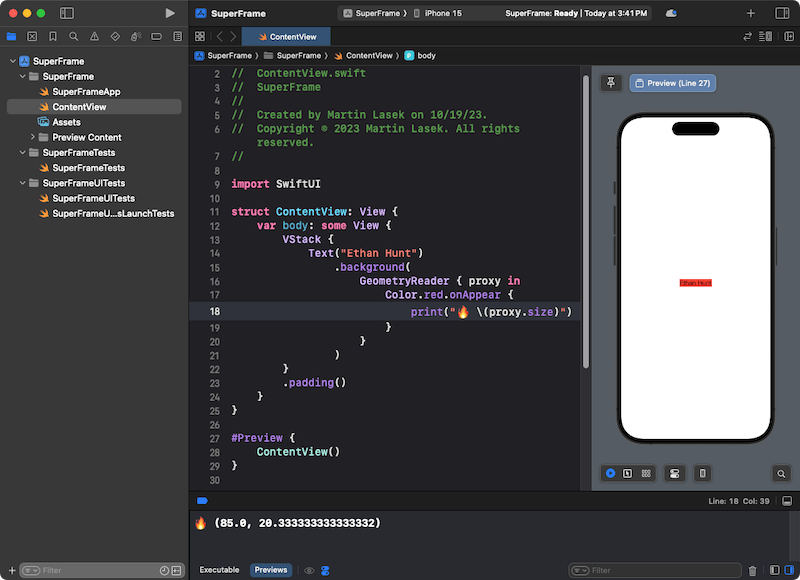
You could've alternatively also just wrapped your Text view with a GeometryReader and do the .onAppear on that Text view instead. But sometimes it's nice to see the color of the frame we are investigating for better visual debugging.
I am happy you read my article and hope you found it useful! If you have any suggestions of any kind don't hesitate let me know. I’d love to hear from you!